Now we go back to Visual Studio. To create a new C++ Plugin we click the Project button in File tab > New > Project. We select the Dewesoft X Plugin Template as our template and fill in the name of our project. After clicking the Ok button a wizard window will appear to guide us through the creation of the plugin.
Since your plugin will be integrated inside Dewesoft, it needs to know Dewesoft's location. We can define the location by ourselves and the installer will create DEWESOFT_EXE_X86 (for 32-bit Dewesoft) and DEWESOFT_EXE_X64 (for 64-bit Dewesoft) system variables for us. In that case, Visual Studio will have to be restarted so it will update its used environmental variables state.
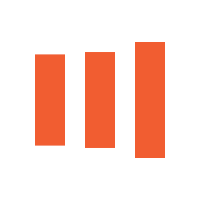
Once those system variables are set, installer will automatically find those fields for you and you will not have to specify the location for further usages.
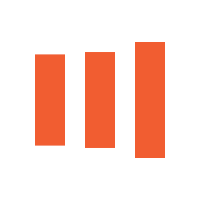
If we want to set those variables ourselves, we can do this using System properties window (it can be found pressing Windows key and searching for Edit the system environment variables), and under advanced tab clicking the Environment variables.
If you only have the 64-bit (or 32-bit) version of Dewesoft on your computer, you will only be able to create 64-bit (or 32-bit) plugins.
After clicking the Next button the following window appears which is used to set Plugin information such as plugin name, its ownership, and version.
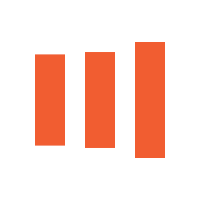
- Plugin name - The name that will be seen in Dewesoft.
- Description - Short description of your plugin.
- Vendor - Company that created the plugin.
- Copyright - Owner of the plugin.
- Major version - Sets the initial major version. The value should change when a breaking change occurs (it's incompatible with previous versions).
- Minor version - Sets the initial minor version. The value should change when new features and bug fixes are added without breaking compatibility.
- Release version - Sets the initial release version. The value should change if the new changes contain only bugfixes.
All fields are optional except for the Plugin name, and they can all be modified later from the code.
After clicking the Next button a final window appears. This window is used to set your Base class name. It is used as a prefix for class and project name. When the Base class name is set, we can click the Finish button and the wizard will generate the plugin template based on your choices.
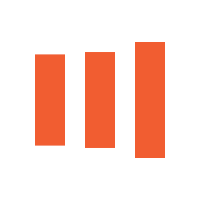
Below the base class name, you can see a dropdown menu, where you can select the example which will be generated for you. There are two options, one is a sine wave example, which will set everything up for outputing a sine wave values to the output channel. The other option is Empty example, which will remove all example code from the project. We recommend using this if you already have some knowledge about Dewesoft plugin development.
The Project name is the name of the file created by the Visual Studio.
The Plugin name is is the name of the plugin as seen in Dewesoft.
The Base class name is the name of the plugin inside of Visual Studio and has to be a valid C++ name.
When a new C++ Plugin project is created, the wizard will create the basic files and project structure needed for development. In the picture below you can see the structure of a project in a tree view with collapsed items. In our case, ProTutorial refers to text, which was used as the Base class name.
Image 7: New C++ Plugin project is created
- proTutorial_latchMath_scalar - Used for communication with Dewesoft through its DCOM interface and creating the Plugin UI.
- proTutorial_latchMath_scalarLib - Your main plugin logic should be defined here. In Example I, we will write plugin logic inside the Plugin project to simplify things.
- proTutorial_latchMath_scalarTest - Contains test cases that will be run during unit testing.
- gtest - Simple library, required by ProTutorialPluginTest for unit testing your plugin. This project should not be modified.
When the solution is built for the first time, we recommend rescanning it (to clear cache). If not, some false positive errors might appear and auto-complete might not work. You can do this by clicking on the Project tab and choosing the Rescan solution from the drop-down list.
Once the plugin is created and you stumble upon Error MSB8036: The Windows SDK version sdk_version was not found. You should retarget your solution to use the one you have installed. You can do this by right clicking on your solution node in Solution explorer and selecting Retarget solution.
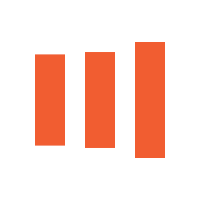
Once the Retarget solution window is opened, you can select "Latest installed version" item.
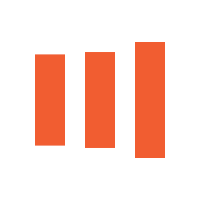
When our project is successfully generated, we will be able to extend Dewesoft. But before implementing the logic behind our plugin, let's take a look at how our plugin is integrated into Dewesoft. In order to do that, we have to start our program using the shortcut F5 or pressing the Start button in the center of Visual Studio's main toolbar.
Image 8: Press the start button in the Visual Studio's main toolbar After Dewesoft loads, our plugin can be accessed in Dewesofts main toolbar in Measure mode under Ch. setup -> ProTutorial. As we can see, it already contains some example elements which were automatically added to the user interface.
Image 9: Elements of newly added plugin
The user interface shown above is defined inside the setup_window.xml file. There are more user interfaces in the project (Setup, Settings), each is found in a different location inside Dewesoft and has a different purpose.
* Setup
- Found in the Dewesoft main toolbar.
- Your main plugin user interface should be defined here.
* Settings
- Found in Options > Settings > Extensions > Plugin.
- General settings, variables, properties, and paths, which are set once and rarely changed should be defined here.
Files associated with them can be found in Visual Studios Solution explorer by expanding ProTutorialPlugin > UI folder.
If your plugin is not visible in Dewesoft you must manually add it. Click the Options icon in the top right corner and from the drop-down list choose Settings. Go to the Extensions tab, click the
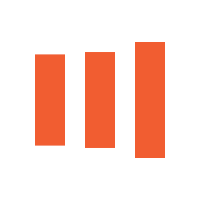
button and find your plugin. Click on it and then click the Enable button on the right.
Image 10: In case the plugin is not visible in Dewesoft X, enable the Latch Math - Scalar under Settings -> Extensions