Before we continue, we need to understand the format of the exported target format. In our simple case, our format requires value-based data as well as single-value channels. Therefore, we will set the export type to etValueBased inside get_ExportType function.
void ExampleWriter::get_ExportType(ExportTypes* Value)
{
*Value = etValueBased;
}
Custom export in this example generates only one file, consisting of the header and the data. File name is obtained in put_filename function, the file stream is created in the StartExport function. Data will be written later. To generate the header part of the file we simply output all the available metadata about the datafile, separated with the new line.
void ExampleWriter::WriteInfoString(BSTR Description, BSTR Value)
{
write_to_output_file(bstr_to_str(Description) + " - " + bstr_to_str(Value), true);
}
This is done in all functions that provide export metadata.
The header content can be seen in the picture below.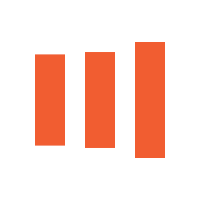
As per the data part of the file, the format requires the first row to contain channel names, while all other rows contain values (each row contains N values, where N is the number of channels). To output the channel names, we use SetChannel function, where we also check for export sample rate to be the same for all channels (which is also a requirement). If the sample rates do not match, the export will exit and nothing will be exported.
To export the data, we use WriteValue function, its body can be seen in the code below. This function is called for each sample, which is written to the file and is later followed with the delimiter or new line (if we just wrote to the last channel). write_to_output_file was implemented in the example and will add string content to the output file.
void ExampleWriter::WriteValue(float Value)
{
write_to_output_file(std::to_string(Value));
sample_counter++;
if (sample_counter % number_of_channels == 0)
{
write_to_output_file("", true);
sample_counter = 0;
}
else
write_to_output_file(get_data_delimiter_char());
}
If we now take a look at the data part of the output file, we can see that the data is organized and supports the Dewesoft format. As you can see, the delimiter is semicolon (";") character because it was selected in the combo box as a default delimiter.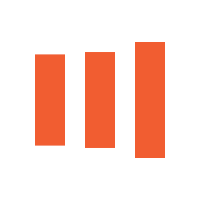
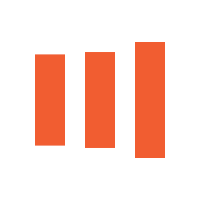
For the purpose of this Pro tutorial, we did not have to modify any Dewesoft internals. But if we wanted to, for example, export any other channel type or stop the export we would need to send a notification to the Dewesoft. For example, if we wanted to export array channels, we notify Dewesoft by returning True when calling the evSupportsArray function. This needs to be done inside DewesoftBridge::OnEvent function. There are many other events that can be used for notifying or sending info to the Dewesoft but they will not be covered in this tutorial.